Introduction to BCrypt Encryption
BCrypt encryption is one of the most powerful and widely used password hashing methods today. With high security features and resistance to brute force attacks, BCrypt has become the top choice for password protection in web applications and information systems. In this article, we will introduce BCrypt in detail, how it works, and why it is important for information security.
How BCrypt Works
Using the Blowfish Algorithm
BCrypt is based on the Blowfish algorithm, a block cipher developed by Bruce Schneier in 1993. Blowfish is known for its fast encryption speed and high security, particularly effective in protecting sensitive data.
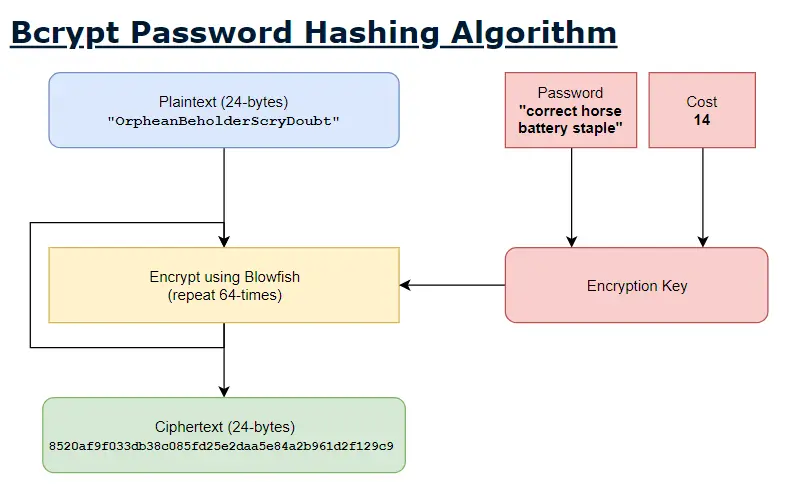
Password Hashing Algorithm
When using BCrypt to hash a password, the process is as follows:
- Generate a salt: A random string of characters is created and combined with the password before hashing. Salt helps prevent rainbow table attacks.
- Apply the hash function: BCrypt applies the Blowfish hash function to the password combined with the salt. This hash function is performed multiple times to increase the difficulty of decryption.
- Store the password: The final result is a string stored in hashed form. This string includes information about the salt and the number of hash iterations.
Benefits of Using BCrypt
Protection Against Brute Force Attacks
One of the biggest benefits of BCrypt is its ability to protect against brute force attacks. By using salt and performing multiple hash iterations, BCrypt makes it extremely time-consuming and resource-intensive to test all password combinations.
Adjustable Difficulty
BCrypt allows adjusting the number of hash iterations, meaning you can increase the difficulty of the hashing process over time. This helps ensure that passwords are always better protected as computers become more powerful.
Compatibility and Ease of Use
BCrypt is widely supported across many programming languages and systems, making it easy for developers to integrate into their applications. Libraries and modules supporting BCrypt are available for most popular languages such as Python, Java, Ruby, and PHP.
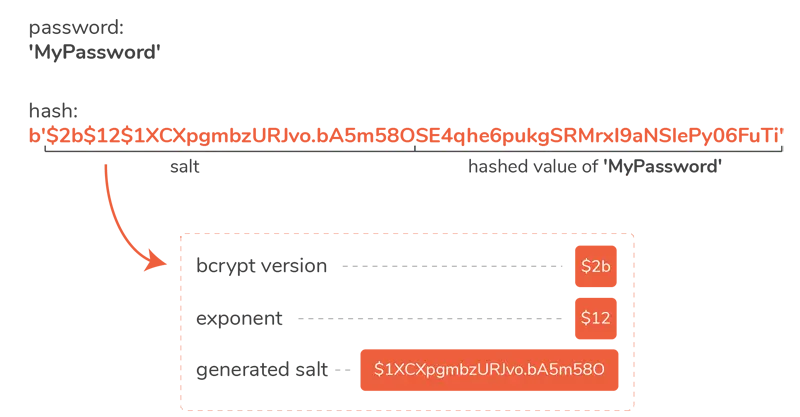
Comparison of BCrypt with Other Hashing Algorithms
BCrypt vs. MD5
MD5 is one of the old and outdated hashing algorithms due to its weak security. MD5 is vulnerable to brute force and collision attacks, making it unsuitable for password protection. In contrast, BCrypt provides much higher security due to its salt usage and adjustable difficulty mechanism.
BCrypt vs. SHA-256
SHA-256 is a strong and widely used hashing algorithm. However, SHA-256 does not have a built-in salt mechanism, making it more vulnerable if not combined with a salt. BCrypt, with its salt usage and adjustable difficulty, remains the preferred choice for password hashing.
BCrypt vs. PBKDF2
PBKDF2 (Password-Based Key Derivation Function 2) is a secure and commonly used password hashing algorithm. PBKDF2 provides salt usage and adjustable hash iterations similar to BCrypt. However, BCrypt is still preferred in many cases due to its flexibility and ease of use.
Implementing BCrypt in Applications
Using BCrypt in Python
To use BCrypt in Python, you can install the bcrypt
library and use the available functions to hash passwords. Here is a basic example of using BCrypt in Python:
import bcrypt
# Hash the password
password = b"mysecretpassword"
hashed = bcrypt.hashpw(password, bcrypt.gensalt())
# Check the password
if bcrypt.checkpw(password, hashed):
print("Password is correct")
else:
print("Password is incorrect")
Using BCrypt in Java
In Java, the BCrypt
library from Spring Security
is a popular choice. Here is an example of using BCrypt in Java:
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
public class BCryptExample {
public static void main(String[] args) {
BCryptPasswordEncoder encoder = new BCryptPasswordEncoder();
String password = "mysecretpassword";
String hashedPassword = encoder.encode(password);
boolean isPasswordMatch = encoder.matches(password, hashedPassword);
System.out.println("Password is correct: " + isPasswordMatch);
}
}
Conclusion
BCrypt is one of the most powerful and secure password hashing methods available today. With its ability to protect against brute force attacks, salt usage, and adjustable difficulty, BCrypt is the top choice for password protection in applications and information systems.
Leave a Reply
View Comments